This tutorial will guide you through building a REST API using Java 17, Spring Boot 3, H2 Database, Spring Data JPA, JWT, Lombok, DTO Pattern, MapStruct, Docker Compose, and Spring Mail. We will use JWT to validate a user during login and registration. Additionally, we will implement account activation via token and other essential features.
Functional Requirements
Register
The user must register an account with their name, DNI, profession, address, country, email, password, and confirm password.
The user must receive an email to activate their account.
The password must include a capital letter, number, and special character.
The email must be in a valid format. After registration, the user needs to check their email to verify the account.
Login
The user must have a valid email and activate their account.
The JWT must be passed in the Bearer Token to process the request.
Activate Account
The user must receive an activation token in their email.
List of Barbers
Search list of barbers.
Update barber details.
Delete barber records.
Search barber by ID.
List of Items
Search for instruments.
Update instruments.
Delete instruments.
Search instruments by ID.
Table of Contents for This Tutorial
- Â Introduction to Spring Boot and its advantages.
- Â Introduction to REST API and DTO Pattern.
- Create our project using Spring Initializer: Spring Initializer.
- Add dependencies in the POM file such as MapStruct and JWT.
- Create our package structure.
- Configure
application.yml
with our database connection and other important properties. - Create our entity class.
- Create our DTO class.
- Create our mapper class.
- Create our security class with the necessary beans.
- Create our configuration class with the necessary beans.
- Add our beans to the Spring Boot application main class.
- Create our Docker file with the configuration to deploy an image and serve mail.
- Create our service and implementation classes.
- Create our repository class.
- Create our exception class.
- Create our controller class.
- Create our constants.
- Create our email HTML template in resources.
Section 1. Introduction to Spring Boot and its advantages.
Spring Boot is an open-source framework that simplifies the development of Spring-based applications. It allows developers to create standalone, production-grade Spring applications with minimal configuration. Spring Boot is designed to streamline the setup and development process by providing a range of pre-configured templates and defaults, thus reducing the complexity and length of the code needed to start a new Spring project.
Advantages of Spring Boot:
Simplified Setup: Spring Boot eliminates the need for complex XML configuration files by using convention over configuration, making it easy to start a new project with minimal setup.
Microservices Support: It is ideal for building microservices, providing a suite of tools and features like embedded servers (Tomcat, Jetty) and metrics, helping developers build and deploy microservices easily.
Production-Ready: Spring Boot includes production-ready features such as metrics, health checks, and externalized configuration, which are crucial for monitoring and managing applications in a production environment.
Embedded Servers: It supports embedded servers, allowing developers to run web applications directly from the Java command line, facilitating simpler deployment processes.
Section 2. Introduction to Rest API and DTO Pattern.
REST API (Representational State Transfer Application Programming Interface)
A REST API is a set of rules and conventions for building and interacting with web services. It uses standard HTTP methods such as GET, POST, PUT, and DELETE to perform CRUD (Create, Read, Update, Delete) operations. REST APIs are stateless, meaning each request from a client to the server must contain all the information needed to understand and process the request. This approach promotes scalability and flexibility, making it a popular choice for building web services.
Advantages of REST API:
1. Simplicity: REST APIs are simple to use and understand, leveraging standard HTTP methods and status codes.
2. Scalability: Statelessness and a clear separation of client and server allow for easy scaling.
3. Flexibility: REST APIs can handle different types of calls, return various data formats, and change structure with the proper versioning.
4. Performance: Lightweight and efficient, REST APIs often result in faster interactions and reduced load on the server.
REST API Best Practices:
1. Nomenclature and Naming Conventions:
– Use nouns to represent resources (e.g., `/users`, `/orders`).
– Use HTTP methods appropriately (e.g., GET for reading, POST for creating).
– Use plural nouns for resource names (e.g., `/books` instead of `/book`).
– Employ lowercase letters and hyphens to separate words (e.g., `/user-profiles`).
2. Common Use:
– GET /users:** Retrieve a list of users.
– POST /users:** Create a new user.
– GET /users/{id}:** Retrieve a specific user by ID.
– PUT /users/{id}:** Update a specific user by ID.
– DELETE /users/{id}:** Delete a specific user by ID.
3. Examples:
HTTP Methods
GET /api/v1/users
POST /api/v1/users
GET /api/v1/users/123
PUT /api/v1/users/123
DELETE /api/v1/users/123
DTO Pattern (Data Transfer Object Pattern)
A DTO (Data Transfer Object) is a design pattern used to transfer data between different layers of an application. DTOs are simple objects that do not contain any business logic but are used to encapsulate data and send it across network boundaries, such as between a client and a server.
Advantages of DTO Pattern:
1. Decoupling: DTOs help decouple the internal representation of data from its external representation, promoting loose coupling.
2. Data Security: By using DTOs, you can control the exposure of sensitive data, sending only what is necessary.
3. Performance Optimization: DTOs can be designed to carry just the data needed for specific operations, reducing the amount of data transferred over the network.
4. Ease of Use: They simplify data binding in different layers, making it easier to manage and use data across the application.
DTO Best Practices:
1. Nomenclature and Naming Conventions:
– Use clear and descriptive names (e.g., `UserDTO`, `OrderDTO`).
– Follow a consistent naming pattern for DTOs (e.g., appending `DTO` to the class name).
2. Common Use:
– UserDTO: Transfer user data between layers without exposing the internal user entity.
– OrderDTO: Transfer order details while encapsulating and hiding the complexity of the order entity.
3. Examples:
public class UserDTO {
private Long id;
private String name;
private String email;
// Getters and Setters
}
public class OrderDTO {
private Long id;
private String product;
private int quantity;
// Getters and Setters
}
Integrating these concepts, we will develop a robust and efficient Spring Boot application that leverages the power of REST APIs and the DTO pattern to provide a seamless experience for managing our barbershop services.
Section 3. Create Our Project Using Spring Initializer
Spring Boot provides a Spring Initializer web tool to quickly create and bootstrap Spring Boot applications. Visit Spring Initializer to generate a new Spring Boot project.
Refer to this screenshot to enter the details to create the Spring Boot project:
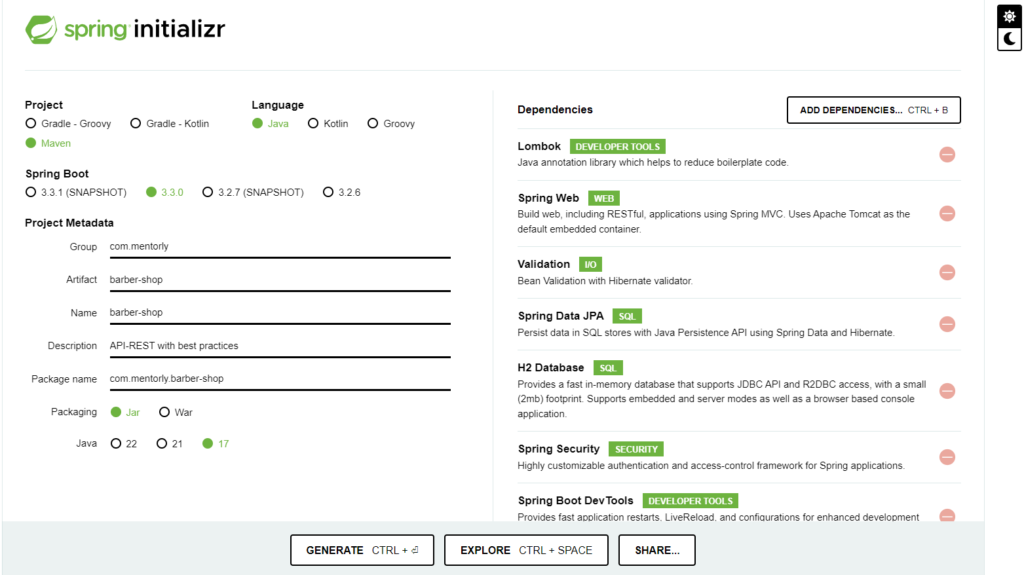
Dependencies to Install:
- Lombok:
- Description: Lombok is a library that helps reduce boilerplate code by generating getters, setters, and other methods at compile time.
- Why We Use It: To simplify our code and improve readability by reducing the need to manually write common methods.
- Spring Web:
- Description: Spring Web is part of the Spring Framework and provides comprehensive support for building web applications, including RESTful services.
- Why We Use It: To create REST endpoints for our application.
- Validation:
- Description: This dependency provides support for bean validation using annotations.
- Why We Use It: To ensure the correctness of the data being processed in our application.
- Spring Data JPA:
- Description: Spring Data JPA simplifies the implementation of data access layers by providing a repository abstraction over JPA.
- Why We Use It: To interact with our database using the JPA (Java Persistence API) specification.
- H2 Database (Driver):
- Description: H2 is an in-memory database engine.
- Why We Use It: To quickly set up a database for development and testing purposes without the need for a full-fledged database server.
- Spring Security:
- Description: Spring Security is a powerful and customizable authentication and access control framework for Java applications.
- Why We Use It: To secure our application by managing user authentication and authorization.
- Spring Boot DevTools:
- Description: Spring Boot DevTools provides features that help improve the development experience, such as automatic restarts and live reloads.
- Why We Use It: To enhance the development workflow by reducing the time needed to apply changes and see the results.
- Java Mail Sender:
- Description: This dependency provides support for sending emails.
- Why We Use It: To send users activation emails and other notifications.
Additionally, we need the JWT dependency for our security configuration. Since it is not available in Spring Initializer, we must manually add it from Maven Central: Maven Central Repository.
JWT (JSON Web Token):
- Description: JWT is a compact, URL-safe means of representing claims to be transferred between two parties. The claims in a JWT are encoded as a JSON object.
- Why We Use It: To implement stateless authentication and authorization by securely transmitting information between parties.
<!--JWT-->
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-api</artifactId>
<version>0.11.5</version>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-impl</artifactId>
<version>0.11.5</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-jackson</artifactId>
<version>0.11.5</version>
<scope>runtime</scope>
</dependency>
Also, We need to add the SpringDoc OpenAPI to the documentation
<!--Springdoc OpenAPI-->
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-starter-webmvc-ui</artifactId>
<version>2.3.0</version>
</dependency>
After Downloading the ZIP File:
- Extract the ZIP File:
- Once you download the ZIP file from Spring Initializer, extract it to your desired location.
- Open the Project in IntelliJ IDEA:
- Open IntelliJ IDEA and select “Open” from the Welcome screen or from the “File” menu.
- Navigate to the extracted project directory and select it to open.
- Load Maven Dependencies:
- After the project opens, IntelliJ IDEA should prompt you to import the Maven project. Click “Load” to allow Maven to download and configure the dependencies.
- If IntelliJ IDEA does not automatically prompt you, right-click on the
pom.xml
file in the project view and select “Maven” > “Reload Project”.
By following these steps, you will have your Spring Boot project set up in IntelliJ IDEA with all the necessary dependencies ready to use. This setup will allow you to start building and developing your application efficiently.
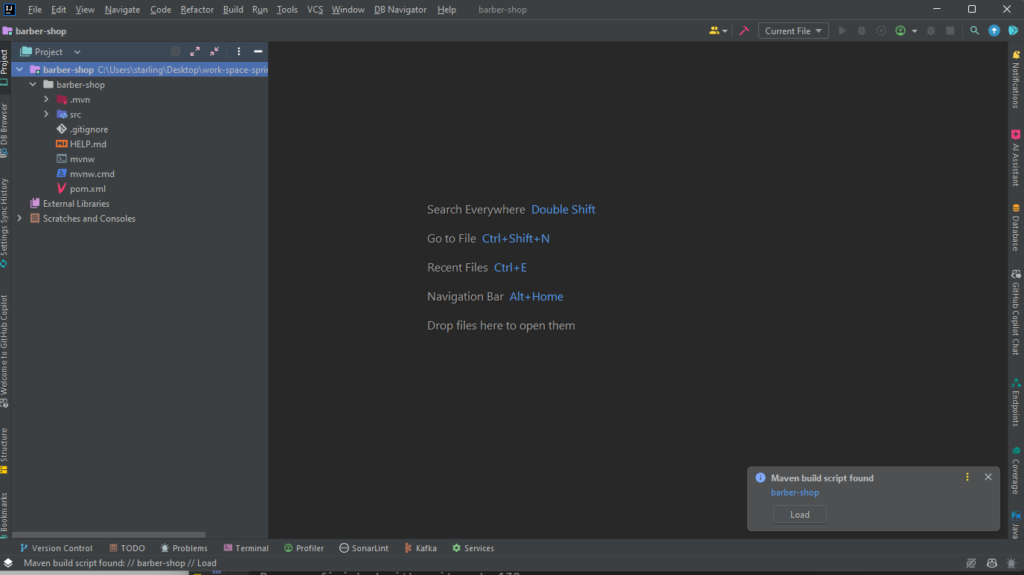
Here is the complete pom.xml
file for our project, including all the necessary dependencies:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>3.3.0</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.mentorly</groupId>
<artifactId>barber-shop</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>barber-shop</name>
<description>API-REST with best practices</description>
<properties>
<java.version>17</java.version>
<org.mapstruct.version>1.5.5.Final</org.mapstruct.version>
</properties>
<dependencies>
<!--Spring Boot Starter Data JPA-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!--Spring Boot Starter Mail-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<!--Spring Security-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
<!--JWT-->
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-api</artifactId>
<version>0.11.5</version>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-impl</artifactId>
<version>0.11.5</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>io.jsonwebtoken</groupId>
<artifactId>jjwt-jackson</artifactId>
<version>0.11.5</version>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-validation</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-devtools</artifactId>
<scope>runtime</scope>
<optional>true</optional>
</dependency>
<!--H2 Database-->
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<!--Springdoc OpenAPI-->
<dependency>
<groupId>org.springdoc</groupId>
<artifactId>springdoc-openapi-starter-webmvc-ui</artifactId>
<version>2.3.0</version>
</dependency>
<!--Mysql Driver-->
<dependency>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct</artifactId>
<version>${org.mapstruct.version}</version>
</dependency>
<!--Lombok-->
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
<dependency>
<groupId>org.springframework.security</groupId>
<artifactId>spring-security-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<pluginManagement>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>${spring-boot.version}</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.8.1</version>
<configuration>
<source>17</source>
<target>17</target>
<annotationProcessorPaths>
<path>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
</path>
<path>
<groupId>org.mapstruct</groupId>
<artifactId>mapstruct-processor</artifactId>
<version>${org.mapstruct.version}</version>
</path>
</annotationProcessorPaths>
</configuration>
</plugin>
</plugins>
</pluginManagement>
</build>
</project>
Integrating these concepts, we will develop a robust and efficient Spring Boot application that leverages the power of REST APIs and the DTO pattern to provide a seamless experience for managing our barbershop services.
FULL STACK BARBER SHOP SPRING BOOT 3 ANGULAR 16
Section 4. Create Our Package Structure
To organize the project properly, we’ll use the following package structure:
config (for configuration classes)
controller (for REST controllers)
dto (for Data Transfer Objects)
entity (for JPA entities)
mapper (for MapStruct mappers)
repository (for Spring Data JPA repositories)
service (for business logic and services)
security (for security-related configuration and JWT classes)
exception (for custom exceptions)
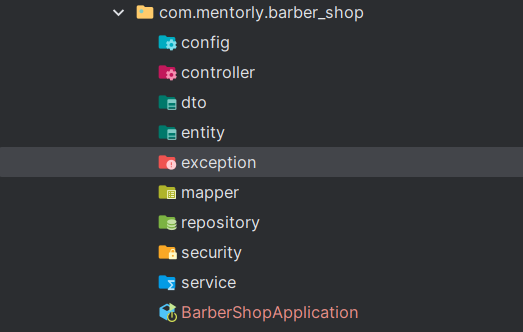
Section 5. Configure application.yml
Configure the database and other essential properties in the src/main/resources/application.yml
file:
spring:
application:
name: Barber-Shop API
datasource:
url: jdbc:h2:mem:testdb
driver-class-name: org.h2.Driver
username: sa
password:
h2:
console:
enabled: true
path: /h2-console
jpa:
hibernate:
ddl-auto: update
show-sql: true
mail:
host: localhost
port: 1025
username: admin
password: admin
properties:
mail:
smtp:
trust: "*"
auth: true
starttls:
enable: true
connection:
timeout: 5000
writetimeout: 5000
security:
jwt:
secret-key: "RSjUBwnNFcjYzUFqFFDw1pCFbfZed5MC2QQVzs+CWeY="
expiration-time: 86400000
logging:
level:
org:
springframework:
security: DEBUG
web: DEBUG
server:
port: 8080
This configures the H2 database, JWT settings, and mail settings for account activation.
Section 6. Create Entity Class
For the User
entity, create the class under the entity
package.
/**
* @author Starling Diaz on ${DATE}.
* @Github https://github.com/NSTLRD
*
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version ${PROJECT_NAME} 1.0
* @since ${DATE}.
*/
package com.mentorly.barber_shop.entity;
import jakarta.persistence.*;
import lombok.*;
import org.springframework.security.core.GrantedAuthority;
import org.springframework.security.core.userdetails.UserDetails;
import javax.security.auth.Subject;
import java.security.Principal;
import java.util.Collection;
import java.util.HashSet;
@Entity
@Table(name = "users")
@Getter
@Setter
@Builder
@AllArgsConstructor
@NoArgsConstructor
public class User implements UserDetails, Principal {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String lastName;
private String dni;
private String profession;
private String address;
private String country;
@Column(unique = true)
private String email;
private String password;
private String token;
private boolean enabled = false; // for account activation
@Override
public Collection<? extends GrantedAuthority> getAuthorities() {
return new HashSet<>();
}
@Override
public String getUsername() {
return email;
}
@Override
public boolean isAccountNonExpired() {
return true;
}
@Override
public boolean isAccountNonLocked() {
return enabled;
}
@Override
public boolean isCredentialsNonExpired() {
return true;
}
@Override
public boolean isEnabled() {
return enabled;
}
public String FullName() {
return name + " " + lastName;
}
}
Section 7. Create DTO Class
We will create the UserDTO
in the dto
package:
/**
* @author Starling Diaz on 10/4/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.dto;
import lombok.*;
@Getter
@Setter
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class UserDTO {
private Long id;
private String name;
private String lastName;
private String dni;
private String profession;
private String address;
private String country;
private String email;
private String password;
}
/**
* @author Starling Diaz on 10/5/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/5/2024.
*/
package com.mentorly.barber_shop.dto;
import jakarta.validation.constraints.Email;
import jakarta.validation.constraints.NotBlank;
import jakarta.validation.constraints.Pattern;
import lombok.Builder;
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
@Builder
public class LoginDTO {
@Email(message = "Invalid email format")
@NotBlank(message = "The email cannot be empty")
@Pattern(regexp = "^[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\\.[a-zA-Z]{2,6}$", message = "Invalid email format")
private String email;
@NotBlank(message = "The password cannot be empty")
@Pattern(regexp = "^(?=.*[0-9])(?=.*[a-zA-Z])(?=\\S+$).{8,}$", message = "The password must have at least 8 characters, including numbers and letters")
private String password;
}
/**
* @author Starling Diaz on 10/5/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/5/2024.
*/
package com.mentorly.barber_shop.dto.response;
import lombok.Builder;
import lombok.Getter;
import lombok.Setter;
@Getter
@Setter
@Builder
public class LoginResponseDTO {
private String token;
}
/**
* @author Starling Diaz on 10/5/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/5/2024.
*/
package com.mentorly.barber_shop.dto.response;
import com.mentorly.barber_shop.entity.User;
import lombok.Getter;
import lombok.NoArgsConstructor;
import lombok.RequiredArgsConstructor;
import lombok.Setter;
import java.time.LocalDateTime;
@Getter
@Setter
@RequiredArgsConstructor
public class UserResponseDTO {
private String id;
private LocalDateTime created;
private LocalDateTime modified;
private LocalDateTime lastLogin;
private String token;
private boolean enabled;
public UserResponseDTO(String id, LocalDateTime created, LocalDateTime modified, LocalDateTime lastLogin, String token, boolean isActive) {
this.id = id;
this.created = created;
this.modified = modified;
this.lastLogin = lastLogin;
this.token = token;
this.enabled = isActive;
}
}
Section 8. Create Mapper Class
Use MapStruct to map between User
and UserDTO
. Create a class in the mapper
package:
package com.mentorly.barber_shop.mapper;
import com.mentorly.barber_shop.dto.UserDTO;
import com.mentorly.barber_shop.entity.User;
import org.mapstruct.Mapper;
import org.mapstruct.factory.Mappers;
@Mapper
public interface UserMapper {
UserMapper INSTANCE = Mappers.getMapper(UserMapper.class);
UserDTO userToUserDTO(User user);
User userDTOToUser(UserDTO userDTO);
}
Section 9. Create Security Configuration
Create the BeanConfig class under the Config package to handle JWT authentication:
This class handles the configuration for common security beans such as AuthenticationProvider
, PasswordEncoder
, AuthenticationManager
, and CorsFilter
. It ensures that we configure the necessary security components for user authentication and CORS handling.
/**
* @author Starling Diaz
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.config;
import lombok.RequiredArgsConstructor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.AuthenticationProvider;
import org.springframework.security.authentication.dao.DaoAuthenticationProvider;
import org.springframework.security.config.annotation.authentication.configuration.AuthenticationConfiguration;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.crypto.bcrypt.BCryptPasswordEncoder;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.web.cors.CorsConfiguration;
import org.springframework.web.cors.UrlBasedCorsConfigurationSource;
import org.springframework.web.filter.CorsFilter;
import java.util.Arrays;
import java.util.Collections;
import static org.springframework.http.HttpHeaders.AUTHORIZATION;
import static org.springframework.http.HttpHeaders.CONTENT_TYPE;
@Configuration
@RequiredArgsConstructor
public class BeanConfig {
private final UserDetailsService userDetailsService;
@Bean
public AuthenticationProvider authenticationProvider() {
DaoAuthenticationProvider authProvider = new DaoAuthenticationProvider();
authProvider.setUserDetailsService(userDetailsService);
authProvider.setPasswordEncoder(passwordEncoder());
return authProvider;
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
@Bean
public AuthenticationManager authenticationManager(AuthenticationConfiguration authConfig) throws Exception {
return authConfig.getAuthenticationManager();
}
@Bean
public CorsFilter corsFilter() {
final UrlBasedCorsConfigurationSource source = new UrlBasedCorsConfigurationSource();
final CorsConfiguration config = new CorsConfiguration();
config.setAllowCredentials(true);
config.setAllowedOrigins(Collections.singletonList("http://localhost:4200"));
config.setAllowedHeaders(Arrays.asList(CONTENT_TYPE, AUTHORIZATION));
config.setAllowedMethods(Arrays.asList("GET", "POST", "PUT", "DELETE"));
source.registerCorsConfiguration("/**", config);
return new CorsFilter(source);
}
}
SecurityConfig Class
This class is responsible for configuring the security filter chain. It specifies which endpoints are publicly accessible and ensures that JWT-based authentication is properly enforced. It also integrates the JWT filter and handles session management.
/**
* @author Starling Diaz
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.config;
import com.mentorly.barber_shop.security.JwFilterServiceSecurity;
import jakarta.servlet.Filter;
import lombok.RequiredArgsConstructor;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.security.authentication.AuthenticationProvider;
import org.springframework.security.config.annotation.method.configuration.EnableMethodSecurity;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.http.SessionCreationPolicy;
import org.springframework.security.web.SecurityFilterChain;
import org.springframework.security.web.authentication.UsernamePasswordAuthenticationFilter;
import static org.springframework.security.config.Customizer.withDefaults;
@Configuration
@EnableWebSecurity
@RequiredArgsConstructor
@EnableMethodSecurity(securedEnabled = true)
public class SecurityConfig {
private final JwFilterServiceSecurity jwtAuthFilter;
private final AuthenticationProvider authenticationProvider;
public static String[] PUBLIC_URLS = {
"/v1/api/**", "/swagger-ui/**", "/h2-console/**",
"/api/v1/users/register", "/api/v1/users/login",
"/api/v1/users/activate-account"
};
@Bean
public SecurityFilterChain securityFilterChain(HttpSecurity http) throws Exception {
http.cors(withDefaults())
.csrf(csrf -> csrf.disable())
.authorizeHttpRequests(authz -> authz
.requestMatchers(PUBLIC_URLS).permitAll()
.anyRequest().authenticated())
.sessionManagement(session -> session.sessionCreationPolicy(SessionCreationPolicy.STATELESS))
.authenticationProvider(authenticationProvider)
.addFilterBefore((Filter) jwtAuthFilter, UsernamePasswordAuthenticationFilter.class);
http.headers().frameOptions().disable(); // For H2 console access
return http.build();
}
}
JwtFilterServiceSecurity Class
This filter checks every request to validate if it contains a valid JWT. If the JWT is valid, it extracts the user information and sets the authentication in the Spring Security context.
/**
* @author Starling Diaz
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.security;
import jakarta.servlet.FilterChain;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
import lombok.RequiredArgsConstructor;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.context.SecurityContextHolder;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.security.core.userdetails.UserDetailsService;
import org.springframework.security.web.authentication.WebAuthenticationDetailsSource;
import org.springframework.web.filter.OncePerRequestFilter;
import java.io.IOException;
import static org.springframework.http.HttpHeaders.AUTHORIZATION;
@Service
@RequiredArgsConstructor
public class JwFilterServiceSecurity extends OncePerRequestFilter {
private final JwTServiceSecurity jwtService;
private final UserDetailsService userDetailsService;
@Override
protected void doFilterInternal(HttpServletRequest request, HttpServletResponse response, FilterChain filterChain)
throws ServletException, IOException {
if (request.getServletPath().contains("/api/v1/users/login") ||
request.getServletPath().contains("/api/v1/users/register") ||
request.getServletPath().contains("/api/v1/users/activate-account")) {
filterChain.doFilter(request, response);
return;
}
final String authHeader = request.getHeader(AUTHORIZATION);
if (authHeader != null && authHeader.startsWith("Bearer ")) {
String jwt = authHeader.substring(7);
String userEmail = jwtService.extractUsername(jwt);
if (userEmail != null && SecurityContextHolder.getContext().getAuthentication() == null) {
UserDetails userDetails = userDetailsService.loadUserByUsername(userEmail);
if (jwtService.validateToken(jwt, userDetails)) {
UsernamePasswordAuthenticationToken authToken = new UsernamePasswordAuthenticationToken(
userDetails, null, userDetails.getAuthorities());
authToken.setDetails(new WebAuthenticationDetailsSource().buildDetails(request));
SecurityContextHolder.getContext().setAuthentication(authToken);
}
}
}
filterChain.doFilter(request, response);
}
}
JwtServiceSecurity Class
This service manages the creation, validation, and extraction of data from the JWT. It handles signing the token and checking its expiration.
/**
* @author Starling Diaz
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.security;
import io.jsonwebtoken.Claims;
import io.jsonwebtoken.Jwts;
import io.jsonwebtoken.io.Decoders;
import io.jsonwebtoken.security.Keys;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.security.core.userdetails.UserDetails;
import org.springframework.stereotype.Service;
import java.security.Key;
import java.util.Date;
import java.util.HashMap;
import java.util.function.Function;
@Service
public class JwTServiceSecurity {
@Value("${security.jwt.secret-key}")
private String secretKey;
@Value("${security.jwt.expiration-time}")
private long jwtExpiration;
public String extractUsername(String token) {
return extractClaim(token, Claims::getSubject);
}
public <T> T extractClaim(String token, Function<Claims, T> claimsResolver) {
final Claims claims = extractAllClaims(token);
return claimsResolver.apply(claims);
}
private Claims extractAllClaims(String token) {
return Jwts.parserBuilder().setSigningKey(getSigningKey()).build().parseClaimsJws(token).getBody();
}
private Key getSigningKey() {
byte[] keyBytes = Decoders.BASE64.decode(secretKey);
return Keys.hmacShaKeyFor(keyBytes);
}
public String generateToken(HashMap<String, Object> claims, UserDetails userDetails) {
return buildToken(claims, userDetails, jwtExpiration);
}
private String buildToken(HashMap<String, Object> claims, UserDetails userDetails, long expiration) {
return Jwts.builder()
.setClaims(claims)
.setSubject(userDetails.getUsername())
.setIssuedAt(new Date(System.currentTimeMillis()))
.setExpiration(new Date(System.currentTimeMillis() + expiration))
.signWith(getSigningKey())
.compact();
}
public boolean validateToken(String token, UserDetails userDetails) {
final String username = extractUsername(token);
return (username.equals(userDetails.getUsername()) && !isTokenExpired(token));
}
private boolean isTokenExpired(String token) {
return extractExpiration(token).before(new Date(System.currentTimeMillis()));
}
private Date extractExpiration(String token) {
return extractClaim(token, Claims::getExpiration);
}
}
Code Order Summary:
- BeanConfig.java: Configures the authentication provider, password encoder, authentication manager, and CORS filter.
- SecurityConfig.java: Defines the security filter chain, session management, and sets up JWT authentication.
- JwFilterServiceSecurity.java: Handles incoming requests by validating the JWT token and authenticating the user if the token is valid.
- JwTServiceSecurity.java: Manages token creation, validation, and extraction of user data from the JWT.
Section 10. Create Service and Implementation Classes
The service layer handles the business logic of the application. Create an interface UserService
and its implementation class UserServiceImpl
in the service
package.
UserService
package com.mentorly.barber_shop.service;
import com.mentorly.barber_shop.dto.LoginDTO;
import com.mentorly.barber_shop.dto.UserDTO;
import com.mentorly.barber_shop.dto.response.LoginResponseDTO;
import com.mentorly.barber_shop.entity.User;
import com.mentorly.barber_shop.exception.TokenExpiredException;
import jakarta.mail.MessagingException;
public interface UserService {
UserDTO registerUser(UserDTO userDTO) throws Exception;
String generateAndSaveActivationToken(User user);
String generateActivationCode(int length);
LoginResponseDTO loginAuthenticate(LoginDTO loginDto);
String activateAccount(String token) throws MessagingException, TokenExpiredException;
}
UserServiceImpl Class
import com.mentorly.barber_shop.constants.EmailTemplateName;
import com.mentorly.barber_shop.dto.LoginDTO;
import com.mentorly.barber_shop.dto.UserDTO;
import com.mentorly.barber_shop.dto.response.LoginResponseDTO;
import com.mentorly.barber_shop.entity.Token;
import com.mentorly.barber_shop.entity.User;
import com.mentorly.barber_shop.exception.TokenExpiredException;
import com.mentorly.barber_shop.exception.UserAlreadyExistsException;
import com.mentorly.barber_shop.exception.UserNotFoundException;
import com.mentorly.barber_shop.mapper.UserMapper;
import com.mentorly.barber_shop.repository.TokenRepository;
import com.mentorly.barber_shop.repository.UserRepository;
import com.mentorly.barber_shop.security.JwTServiceSecurity;
import com.mentorly.barber_shop.service.UserService;
import jakarta.mail.MessagingException;
import lombok.AllArgsConstructor;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.security.authentication.AuthenticationManager;
import org.springframework.security.authentication.UsernamePasswordAuthenticationToken;
import org.springframework.security.core.AuthenticationException;
import org.springframework.security.crypto.password.PasswordEncoder;
import org.springframework.stereotype.Service;
import java.security.SecureRandom;
import java.time.LocalDateTime;
import java.util.HashMap;
import java.util.Optional;
@Service
@AllArgsConstructor
public class UserServiceImpl implements UserService {
private final UserRepository userRepository;
private final PasswordEncoder passwordEncoder;
private final TokenRepository tokenRepository;
private final EmailServiceImpl emailServiceImpl;
private final AuthenticationManager authenticationManager;
private final JwTServiceSecurity jwTServiceSecurity;
@Value("${mailing.frontend.activation.activationUrl}")
private String activationUrl;
@Override
public UserDTO registerUser(UserDTO userDTO) throws Exception {
if(userRepository.findByEmail(userDTO.getEmail()).isPresent()){
throw new UserAlreadyExistsException("User with email: " + userDTO.getEmail() + " already exists");
}
// Map DTO to entity
User user = UserMapper.INSTANCE.userDTOToUser(userDTO);
// Encrypt the password before saving
user.setPassword(passwordEncoder.encode(user.getPassword()));
user.setEnabled(false); // Account is not enabled until activation
// Save user in the database
userRepository.save(user);
//send the verification email
sendVerificationEmail(user);
return UserMapper.INSTANCE.userToUserDTO(user);
}
private void sendVerificationEmail(User user) throws MessagingException {
//generate new token
var newToken = generateAndSaveActivationToken(user);
user.setToken(newToken);
userRepository.save(user);
//send email
emailServiceImpl.sendEmail(
user.getEmail(),
user.FullName(),
EmailTemplateName.ACTIVATE_ACCOUNT,
activationUrl,
newToken,
"Activate your account"
);
}
@Override
public String generateAndSaveActivationToken(User user) {
String generatedToken = generateActivationCode(6);
var token = Token.builder()
.token(generatedToken)
.createdAt(LocalDateTime.now())
.expiredAt(LocalDateTime.now().plusMinutes(45))
.user(user)
.build();
tokenRepository.save(token);
return generatedToken;
}
@Override
public String generateActivationCode(int length) {
String characters = "123456789";
StringBuilder result = new StringBuilder();
SecureRandom random = new SecureRandom();
for (int i = 0; i < length; i++) {
int randomIndex = random.nextInt(characters.length());
result.append(characters.charAt(randomIndex));
}
return result.toString();
}
@Override
public LoginResponseDTO loginAuthenticate(LoginDTO loginDto) {
if(loginDto.getEmail() == null || loginDto.getEmail().isEmpty()){
throw new RuntimeException("Email is required");
}
if(loginDto.getPassword() == null || loginDto.getPassword().isEmpty()){
throw new RuntimeException("Password is required");
}
try {
var auth = authenticationManager.authenticate(
new UsernamePasswordAuthenticationToken(loginDto.getEmail(), loginDto.getPassword())
);
var user = (User) auth.getPrincipal();
var claims = new HashMap<String, Object>();
claims.put("name", user.getName());
claims.put("email", user.getEmail());
claims.put("id", user.getId());
var jwtToken = jwTServiceSecurity.generateToken(claims, user);
return LoginResponseDTO.builder().token(jwtToken).build();
} catch (AuthenticationException e){
if(userRepository.findByEmail(loginDto.getEmail()).isPresent()){
throw new RuntimeException("Password is incorrect");
} else {
throw new UserNotFoundException("No user found with the provided email address");
}
}
}
@Override
public String activateAccount(String token) throws MessagingException, TokenExpiredException {
Optional<Token> tokenOptional = Optional.ofNullable(tokenRepository.findByToken(token));
if(!tokenOptional.isPresent()){
throw new TokenExpiredException("Invalid Token");
}
Token savedToken = tokenOptional.get();
if(LocalDateTime.now().isAfter(savedToken.getExpiredAt())){
sendVerificationEmail(savedToken.getUser());
throw new TokenExpiredException("Activation token has expired. A new token has been sent to the email address.");
}
User user = savedToken.getUser();
if (user == null) {
throw new UserNotFoundException("User not found");
}
user.setEnabled(true);
userRepository.save(user);
savedToken.setValidatedAt(LocalDateTime.now());
tokenRepository.save(savedToken);
return token;
}
}
Section 11. Create Repository Class
The repository layer will interact with the database. Create the UserRepository
interface in the repository
package:
UserRepository Interfaces:
import com.mentorly.barber_shop.entity.User;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import java.util.Optional;
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
Optional<User> findByEmail(String email);
}
TokenRepository Interfaces:
@Repository
public interface TokenRepository extends JpaRepository<Token, Integer> {
Token findByToken(String token);
}
Section 12. Create Exception Class
To handle custom exceptions, create a ExceptionResponse,GlobalException
Handler, IncorrectPasswordException, TokenExpiredException, UserAlreadyExistsException, UserNotFoundException classes in the exception
package:
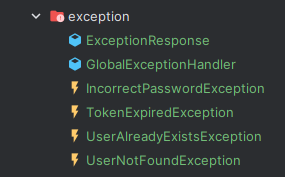
ExceptionResponse
/**
* @author Starling Diaz on 10/4/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.exception;
import com.fasterxml.jackson.annotation.JsonInclude;
import lombok.*;
import java.time.LocalDateTime;
@Getter
@Setter
@Builder
@AllArgsConstructor
@NoArgsConstructor
@JsonInclude(JsonInclude.Include.NON_EMPTY)
public class ExceptionResponse {
private LocalDateTime timestamp;
private int status;
private String error;
private String messageError;
private String path;
}
GlobalExceptionHandle
/**
* @author Starling Diaz on 10/4/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.exception;
import jakarta.servlet.http.HttpServletRequest;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.FieldError;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import java.time.LocalDateTime;
import java.util.HashMap;
import java.util.Map;
@ControllerAdvice
public class GlobalExceptionHandler {
private static final Logger log = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(MethodArgumentNotValidException.class)
public ResponseEntity<ExceptionResponse> handleValidationExceptions(MethodArgumentNotValidException ex, HttpServletRequest request) {
Map<String, String> errors = new HashMap<>();
ex.getBindingResult().getAllErrors().forEach(error -> {
String fieldName = ((FieldError) error).getField();
String errorMessage = error.getDefaultMessage();
errors.put(fieldName, errorMessage);
});
ExceptionResponse response = ExceptionResponse.builder()
.timestamp(LocalDateTime.now())
.status(HttpStatus.BAD_REQUEST.value())
.error("Bad Request")
.messageError(String.join("; ", errors.values()))
.path(request.getRequestURI())
.build();
return new ResponseEntity<>(response, HttpStatus.BAD_REQUEST);
}
@ExceptionHandler({UserNotFoundException.class, IncorrectPasswordException.class, IllegalArgumentException.class})
public ResponseEntity<ExceptionResponse> handleAuthenticationExceptions(RuntimeException ex, HttpServletRequest request) {
HttpStatus status = HttpStatus.UNAUTHORIZED;
if (ex instanceof UserNotFoundException) {
status = HttpStatus.NOT_FOUND;
} else if (ex instanceof IllegalArgumentException) {
status = HttpStatus.BAD_REQUEST;
}
ExceptionResponse response = ExceptionResponse.builder()
.timestamp(LocalDateTime.now())
.status(status.value())
.error(status.getReasonPhrase())
.messageError(ex.getMessage())
.path(request.getRequestURI())
.build();
return new ResponseEntity<>(response, status);
}
@ExceptionHandler(Exception.class)
public ResponseEntity<ExceptionResponse> handleGlobalException(Exception ex, HttpServletRequest request) {
ExceptionResponse response = ExceptionResponse.builder()
.timestamp(LocalDateTime.now())
.status(HttpStatus.INTERNAL_SERVER_ERROR.value())
.error("Internal Server Error")
.messageError(ex.getMessage())
.path(request.getRequestURI())
.build();
return new ResponseEntity<>(response, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
IncorrectPasswordException
/**
* @author Starling Diaz on 6/2/2024.
* @Academy mentorly
* @version bank-stark 1.0
* @since 6/2/2024.
*/
package com.mentorly.barber_shop.exception;
public class IncorrectPasswordException extends RuntimeException {
public IncorrectPasswordException(String message) {
super(message);
}
}
TokenExpiredException
/**
* @author Starling Diaz on 6/2/2024.
* @Academy mentorly
* @version bank-stark 1.0
* @since 6/2/2024.
*/
package com.mentorly.barber_shop.exception;
public class TokenExpiredException extends Exception{
public TokenExpiredException(String message) {
super(message);
}
}
UserAlreadyExistsException
/**
* @author Starling Diaz on 10/4/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/4/2024.
*/
package com.mentorly.barber_shop.exception;
public class UserAlreadyExistsException extends RuntimeException{
public UserAlreadyExistsException(String message) {
super(message);
}
}
UserNotFoundException
/**
* @author Starling Diaz on 6/2/2024.
* @Academy mentorly
* @version bank-stark 1.0
* @since 6/2/2024.
*/
package com.mentorly.barber_shop.exception;
public class UserNotFoundException extends RuntimeException {
public UserNotFoundException(String message) {
super(message);
}
}
Section 14. Create Docker File to Email Service
To send emails, you can use a maildev service l. Create a docker-compose.ym
l
file at the root of your project:
version: '3'
services:
app:
build: .
ports:
- "8080:8080"
mail-dev:
container_name: mail-dev-barbershop
image: maildev/maildev
ports:
- "1080:1080"
- "1025:1025"
Run the command docker compose up
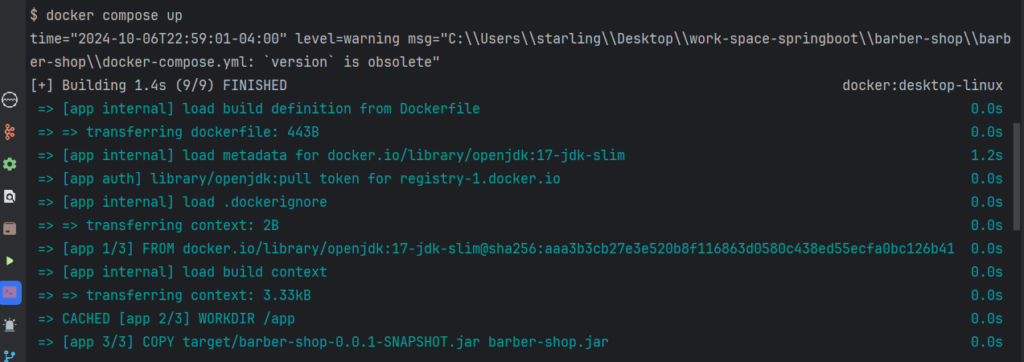
Section 15. Create Controller Class
The controller will handle HTTP requests. Create UserController under the controller
package to handle registration, login, and account activation.
/**
* @author Starling Diaz on 10/5/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/5/2024.
*/
package com.mentorly.barber_shop.controller;
import com.mentorly.barber_shop.dto.LoginDTO;
import com.mentorly.barber_shop.dto.UserDTO;
import com.mentorly.barber_shop.dto.response.LoginResponseDTO;
import com.mentorly.barber_shop.dto.response.UserResponseDTO;
import com.mentorly.barber_shop.exception.IncorrectPasswordException;
import com.mentorly.barber_shop.exception.TokenExpiredException;
import com.mentorly.barber_shop.exception.UserNotFoundException;
import com.mentorly.barber_shop.service.UserService;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.tags.Tag;
import jakarta.mail.MessagingException;
import jakarta.validation.Valid;
import lombok.RequiredArgsConstructor;
import org.springframework.http.HttpStatus;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/v1/users")
@Tag(name = "User Controller", description = "Manage user accounts")
@RequiredArgsConstructor
public class UserController {
private final UserService userService;
@PostMapping("/register")
@Operation(summary = "Register a new user", description = "Registers a new user and provides a JWT for authentication.")
@ApiResponse(responseCode = "201", description = "User registered successfully")
@ApiResponse(responseCode = "400", description = "Email already registered")
@ApiResponse(responseCode = "500", description = "Internal server error")
public ResponseEntity<?> registerUser(@RequestBody @Valid UserDTO userDto) throws Exception {
UserDTO userResponse = userService.registerUser(userDto);
return new ResponseEntity<>(userResponse, HttpStatus.CREATED);
}
@PostMapping("/login")
@Operation(summary = "User login", description = "Authenticates a user and issues a JWT.")
@ApiResponse(responseCode = "200", description = "User logged in successfully")
@ApiResponse(responseCode = "401", description = "Incorrect credentials")
@ApiResponse(responseCode = "404", description = "User not found")
@ApiResponse(responseCode = "500", description = "Internal server error")
public ResponseEntity<LoginResponseDTO> loginUser(@Valid @RequestBody LoginDTO loginDto) {
try {
LoginResponseDTO loginResponse = userService.loginAuthenticate(loginDto);
return ResponseEntity.ok(loginResponse);
} catch (UserNotFoundException | IncorrectPasswordException ex) {
throw ex;
} catch (Exception ex) {
throw new RuntimeException("Internal Server Error", ex);
}
}
@GetMapping("/activate-account")
@Operation(summary = "Activate account", description = "Activate user account")
@ApiResponse(responseCode = "200", description = "Account activated successfully")
@ApiResponse(responseCode = "400", description = "Bad request")
@ApiResponse(responseCode = "500", description = "Internal server error")
public ResponseEntity<?> activateAccount(@RequestParam String token) throws TokenExpiredException, MessagingException {
userService.activateAccount(token);
return ResponseEntity.ok("Account activated successfully");
}
}
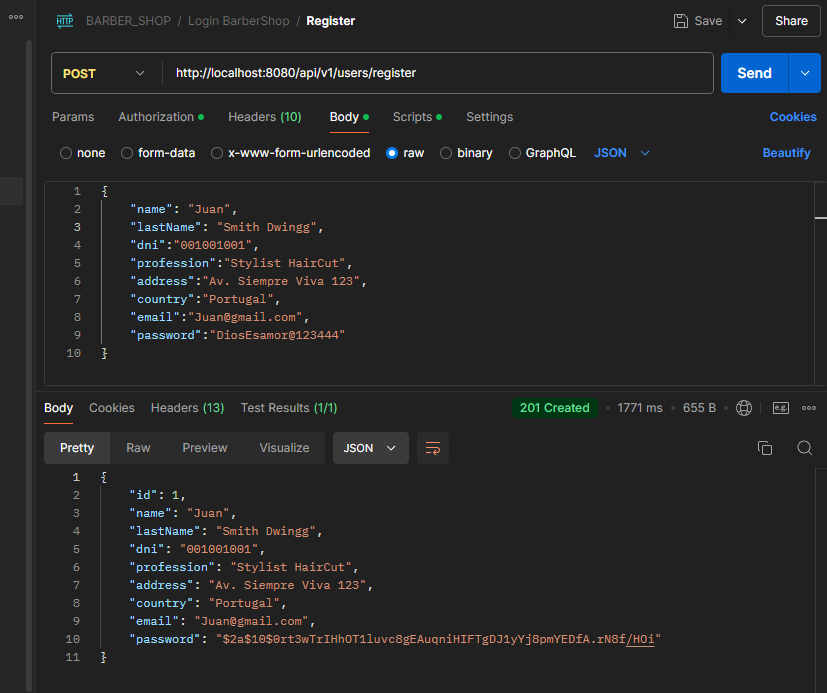
And We Need verified the token that we received in our gmail.
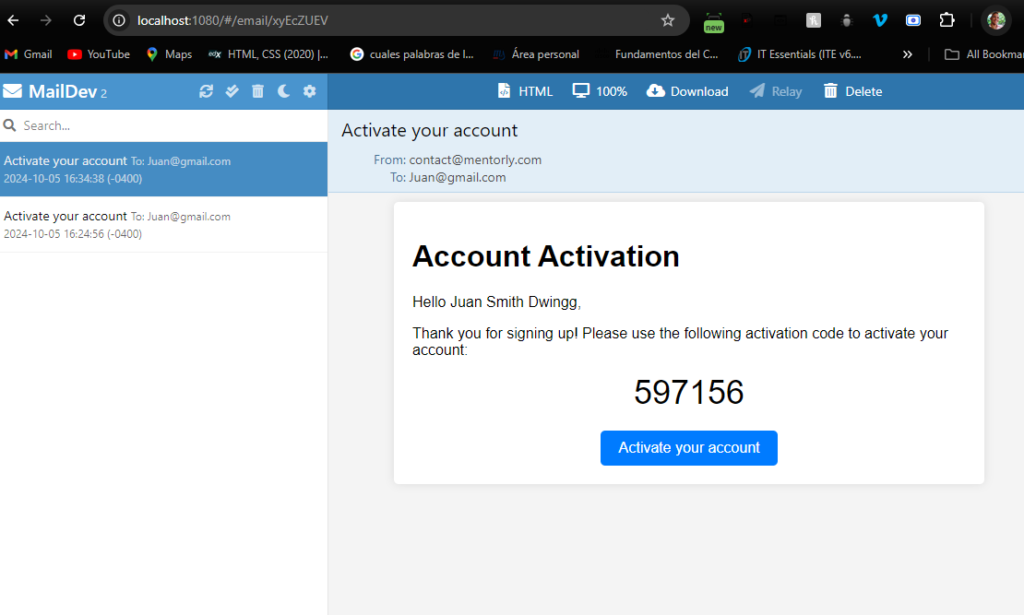
Put the code into the endpoint to active the account
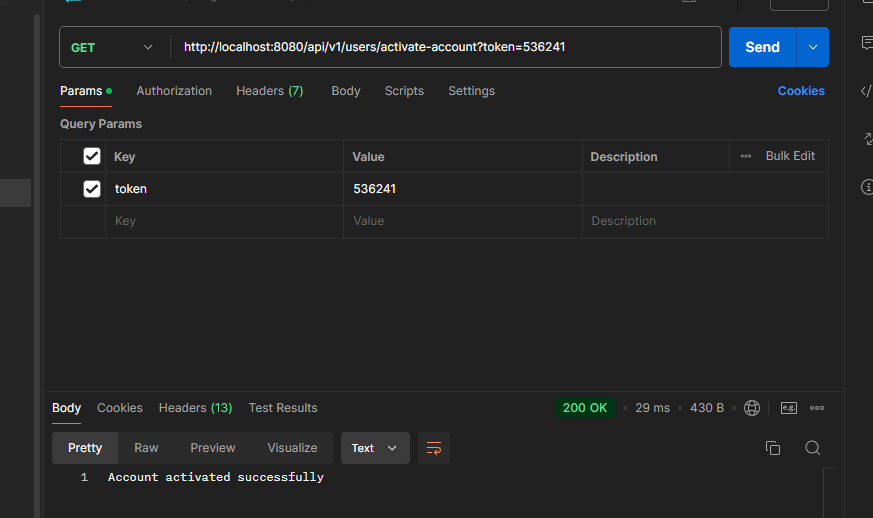
in case you dont see the token into the gmail you can going to the http://localhost:8080/h2-console is the UI to h2 console. and login with user sa and then connect, is no necesarry put password.
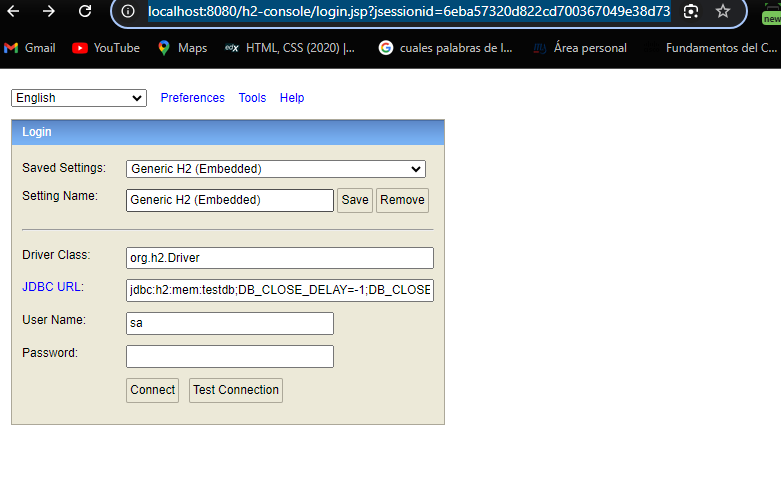
Select the user table, and find the token
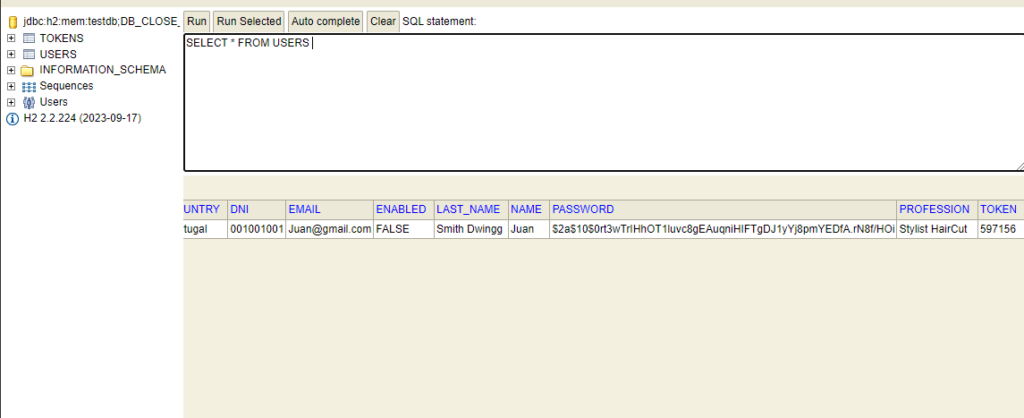
Section 16. Create functionalities Barber.
List of Barbers
We will implement four functionalities:
- Search list of barbers (GET
/barbers
) - Update barber details (PUT
/barbers/{id}
) - Delete barber records (DELETE
/barbers/{id}
) - Search barber by ID (GET
/barbers/{id}
)
Barber Entity
@Data
@Entity
@Table(name = "barbers")
public class Barber {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String experience;
private String specialty;
}
BarberDTO
@Getter
@Setter
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class BarberDTO {
private Long id;
private String name;
private String experience;
private String specialty;
}
BarberRepository
@Repository
public interface BarberRepository extends JpaRepository<Barber, Long> {
}
BarberService Interface
public interface BarberService {
List<BarberDTO> getAllBarbers();
BarberDTO getBarberById(Long id);
BarberDTO updateBarber(Long id, BarberDTO barberDTO);
BarberDTO createBarber(BarberDTO barberDTO); // New method for creating a barber
void deleteBarber(Long id);
}
BarberServiceImpl
@Service
@AllArgsConstructor
public class BarberServiceImpl implements BarberService {
private BarberRepository barberRepository;
private BarberMapper barberMapper;
@Override
public List<BarberDTO> getAllBarbers() {
return barberRepository.findAll()
.stream()
.map(barberMapper::barberToBarberDTO)
.collect(Collectors.toList());
}
@Override
public BarberDTO getBarberById(Long id) {
Barber barber = barberRepository.findById(id)
.orElseThrow(() -> new BarberNotFoundException("Barber not found"));
return barberMapper.barberToBarberDTO(barber);
}
@Override
public BarberDTO updateBarber(Long id, BarberDTO barberDTO) {
Barber existingBarber = barberRepository.findById(id)
.orElseThrow(() -> new BarberNotFoundException("Barber not found"));
barberMapper.barberDTOToBarber(barberDTO); // Using mapper to map DTO to entity
existingBarber.setName(barberDTO.getName());
existingBarber.setExperience(barberDTO.getExperience());
existingBarber.setSpecialty(barberDTO.getSpecialty());
Barber updatedBarber = barberRepository.save(existingBarber);
return barberMapper.barberToBarberDTO(updatedBarber);
}
@Override
public BarberDTO createBarber(BarberDTO barberDTO) {
Barber barber = barberMapper.barberDTOToBarber(barberDTO); // Map DTO to entity
Barber savedBarber = barberRepository.save(barber); // Save entity to database
return barberMapper.barberToBarberDTO(savedBarber); // Map saved entity back to DTO
}
@Override
public void deleteBarber(Long id) {
barberRepository.deleteById(id);
}
}
BarberController
@RestController
@RequestMapping("/api/v1/barbers")
public class BarberController {
@Autowired
private BarberService barberService;
@GetMapping
public ResponseEntity<List<BarberDTO>> getAllBarbers() {
return ResponseEntity.ok(barberService.getAllBarbers());
}
@GetMapping("/{id}")
public ResponseEntity<BarberDTO> getBarberById(@PathVariable Long id) {
return ResponseEntity.ok(barberService.getBarberById(id));
}
@PostMapping
public ResponseEntity<BarberDTO> createBarber(@RequestBody BarberDTO barberDTO) {
return ResponseEntity.ok(barberService.createBarber(barberDTO));
}
@PutMapping("/{id}")
public ResponseEntity<BarberDTO> updateBarber(@PathVariable Long id, @RequestBody BarberDTO barberDTO) {
return ResponseEntity.ok(barberService.updateBarber(id, barberDTO));
}
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteBarber(@PathVariable Long id) {
barberService.deleteBarber(id);
return ResponseEntity.noContent().build();
}
}
Section 17. Create functionalities ItemBarber
List of Enpoints for ItemBarber:
- Search list of ItemBarbers (GET
/item-barbers
) - Search ItemBarber by ID (GET
/item-barbers/{id}
) - Create a new ItemBarber (POST
/item-barbers
) - Update ItemBarber details (PUT
/item-barbers/{id}
) - Delete ItemBarber records (DELETE
/item-barbers/{id}
)
Step 1: Create ItemBarberController.java
This class will manage the REST API endpoints for handling CRUD operations for ItemBarber
.
package com.mentorly.barber_shop.controller;
import com.mentorly.barber_shop.dto.ItemBarberDTO;
import com.mentorly.barber_shop.service.ItemBarberService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.ResponseEntity;
import org.springframework.web.bind.annotation.*;
import java.util.List;
@RestController
@RequestMapping("/api/v1/item-barbers")
public class ItemBarberController {
@Autowired
private ItemBarberService itemBarberService;
// Search list of ItemBarbers
@GetMapping
public ResponseEntity<List<ItemBarberDTO>> getAllItemBarbers() {
return ResponseEntity.ok(itemBarberService.getAllItemBarbers());
}
// Search ItemBarber by ID
@GetMapping("/{id}")
public ResponseEntity<ItemBarberDTO> getItemBarberById(@PathVariable Long id) {
return ResponseEntity.ok(itemBarberService.getItemBarberById(id));
}
// Create a new ItemBarber
@PostMapping
public ResponseEntity<ItemBarberDTO> createItemBarber(@RequestBody ItemBarberDTO itemBarberDTO) {
return ResponseEntity.ok(itemBarberService.createItemBarber(itemBarberDTO));
}
// Update ItemBarber details
@PutMapping("/{id}")
public ResponseEntity<ItemBarberDTO> updateItemBarber(@PathVariable Long id, @RequestBody ItemBarberDTO itemBarberDTO) {
return ResponseEntity.ok(itemBarberService.updateItemBarber(id, itemBarberDTO));
}
// Delete ItemBarber records
@DeleteMapping("/{id}")
public ResponseEntity<Void> deleteItemBarber(@PathVariable Long id) {
itemBarberService.deleteItemBarber(id);
return ResponseEntity.noContent().build();
}
}
Step 2: Create ItemBarberService.java
This is the service interface that will define the methods to manage ItemBarber
.
package com.mentorly.barber_shop.service;
import com.mentorly.barber_shop.dto.ItemBarberDTO;
import java.util.List;
public interface ItemBarberService {
List<ItemBarberDTO> getAllItemBarbers();
ItemBarberDTO getItemBarberById(Long id);
ItemBarberDTO createItemBarber(ItemBarberDTO itemBarberDTO);
ItemBarberDTO updateItemBarber(Long id, ItemBarberDTO itemBarberDTO);
void deleteItemBarber(Long id);
}
Step 3: Create ItemBarberServiceImpl.java
This is the implementation of the ItemBarberService
interface. It will contain the business logic for managing ItemBarber
.
package com.mentorly.barber_shop.service.impl;
import com.mentorly.barber_shop.dto.ItemBarberDTO;
import com.mentorly.barber_shop.entity.ItemBarber;
import com.mentorly.barber_shop.exception.ItemBarberNotFoundException;
import com.mentorly.barber_shop.mapper.ItemBarberMapper;
import com.mentorly.barber_shop.repository.ItemBarberRepository;
import com.mentorly.barber_shop.service.ItemBarberService;
import lombok.AllArgsConstructor;
import org.springframework.stereotype.Service;
import java.util.List;
import java.util.stream.Collectors;
@Service
@AllArgsConstructor
public class ItemBarberServiceImpl implements ItemBarberService {
private ItemBarberRepository itemBarberRepository;
private ItemBarberMapper itemBarberMapper;
@Override
public List<ItemBarberDTO> getAllItemBarbers() {
return itemBarberRepository.findAll()
.stream()
.map(itemBarberMapper::itemBarberToItemBarberDTO)
.collect(Collectors.toList());
}
@Override
public ItemBarberDTO getItemBarberById(Long id) {
ItemBarber itemBarber = itemBarberRepository.findById(id)
.orElseThrow(() -> new ItemBarberNotFoundException("ItemBarber not found"));
return itemBarberMapper.itemBarberToItemBarberDTO(itemBarber);
}
@Override
public ItemBarberDTO createItemBarber(ItemBarberDTO itemBarberDTO) {
ItemBarber itemBarber = itemBarberMapper.itemBarberDTOToItemBarber(itemBarberDTO);
ItemBarber savedItemBarber = itemBarberRepository.save(itemBarber);
return itemBarberMapper.itemBarberToItemBarberDTO(savedItemBarber);
}
@Override
public ItemBarberDTO updateItemBarber(Long id, ItemBarberDTO itemBarberDTO) {
ItemBarber existingItemBarber = itemBarberRepository.findById(id)
.orElseThrow(() -> new ItemBarberNotFoundException("ItemBarber not found"));
existingItemBarber.setName(itemBarberDTO.getName());
existingItemBarber.setSpecialty(itemBarberDTO.getSpecialty());
existingItemBarber.setYearsOfExperience(itemBarberDTO.getYearsOfExperience());
ItemBarber updatedItemBarber = itemBarberRepository.save(existingItemBarber);
return itemBarberMapper.itemBarberToItemBarberDTO(updatedItemBarber);
}
@Override
public void deleteItemBarber(Long id) {
itemBarberRepository.deleteById(id);
}
Step 4: Create ItemBarberDTO.java
This is the Data Transfer Object (DTO) for ItemBarber
.
package com.mentorly.barber_shop.dto;
import lombok.*;
@Getter
@Setter
@Builder
@NoArgsConstructor
@AllArgsConstructor
public class ItemBarberDTO {
private Long id;
private String name;
private String specialty;
private int yearsOfExperience;
}
Step 5: Create ItemBarberMapper.java
This is the mapper interface that converts between the ItemBarber
entity and the ItemBarberDTO
.
package com.mentorly.barber_shop.mapper;
import com.mentorly.barber_shop.dto.ItemBarberDTO;
import com.mentorly.barber_shop.entity.ItemBarber;
import org.mapstruct.Mapper;
import org.mapstruct.factory.Mappers;
@Mapper(componentModel = "spring")
public interface ItemBarberMapper {
ItemBarberMapper INSTANCE = Mappers.getMapper(ItemBarberMapper.class);
ItemBarberDTO itemBarberToItemBarberDTO(ItemBarber itemBarber);
ItemBarber itemBarberDTOToItemBarber(ItemBarberDTO itemBarberDTO);
}
Step 6: Create ItemBarberRepository.java
This repository interface will handle all database operations for the ItemBarber
entity.
package com.mentorly.barber_shop.repository;
import com.mentorly.barber_shop.entity.ItemBarber;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
@Repository
public interface ItemBarberRepository extends JpaRepository<ItemBarber, Long> {
}
Step 7: Create ItemBarber.java (Entity)
You need to define the ItemBarber
entity that will map to the database.
package com.mentorly.barber_shop.entity;
import lombok.*;
import javax.persistence.*;
@Entity
@Table(name = "item_barbers")
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
public class ItemBarber {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String specialty;
private int yearsOfExperience;
}
Step 8: Create Custom Exception.
You can create a custom exception for when an ItemBarber
is not found.
/**
* @author Starling Diaz on 10/6/2024.
* @Github https://github.com/NSTLRD
* @Website https://mentorly.blog/
* @Academy https://www.mentor-ly.com/
* @version barber-shop 1.0
* @since 10/6/2024.
*/
package com.mentorly.barber_shop.exception;
public class ItemNotFoundException extends RuntimeException {
public ItemNotFoundException(String message) {
super(message);
}
}
Conclusion
Thank you for following along with the development of the Barber Shop API project! We hope that this guide has been helpful in understanding the process of building a Spring Boot REST API with best practices. Feel free to explore the full project on GitHub and stay connected through our various platforms to continue learning and improving your skills.
For more projects, tutorials, and resources, check out the links below:
Contact and Social Links
- GitHub: Starling Diaz
- Website: Mentorly Blog
- LinkedIn: LinkedIn
- Mentorly Academy: Mentorly Academy
- YouTube: Mentorly YouTube
Feel free to contribute to the repository, share your feedback, or reach out if you have any questions. We’re looking forward to connecting with you across our platforms. Happy coding!